Canvas generative art
JS Canvas is a little tricky to get your head around at first but once you've got the basics down it's a lot of fun to play around.
I've been working on some generative art code and one of the best decisions I made was to only use predictable random numbers throughout. This means that you can feed a single seed integer into the algorithm and you get the same artwork out each time. This is a really nice property of an algorithm if you're playing and debugging a lot and if you really need properly random numbers for production you can switch out the random numbers code later.
I found this pseudo-random number algorithm online which produces numbers that jump about enough to look random, without actually being random at all. It implements the JS Generators syntax, which is very handy.
export function* pseudoRandom(seed) {
let value = seed;
while (true) {
value = (value * 16807) % 2147483647;
yield value;
}
}
First generative art evolution
I haven't saved every version of the code each step of the way but I enjoy looking back through the renders to see how it's progressed over the hours. Enjoy!
Start
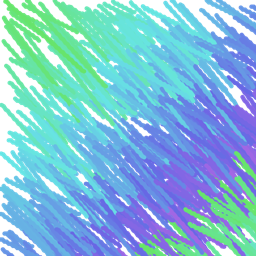
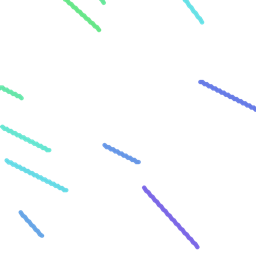
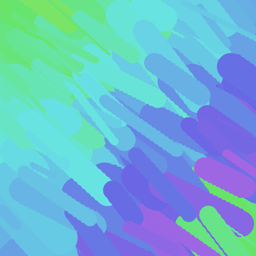
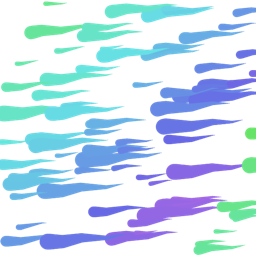
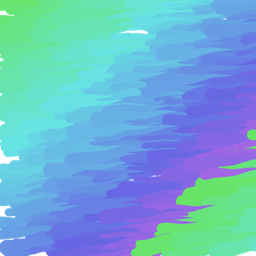
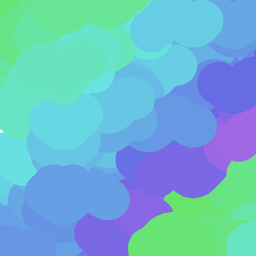
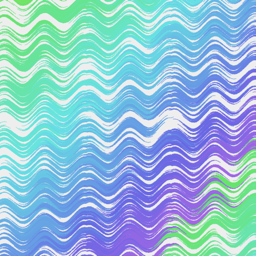
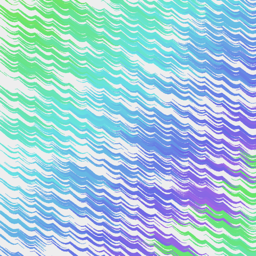
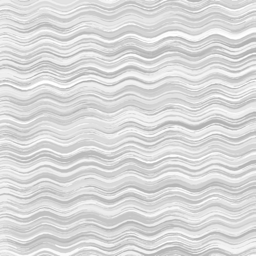
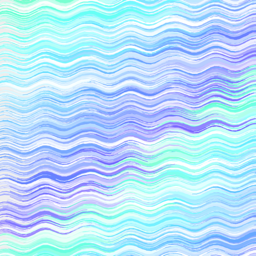
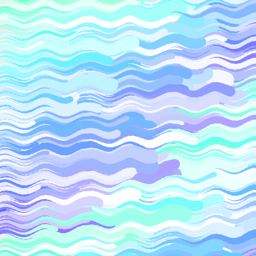
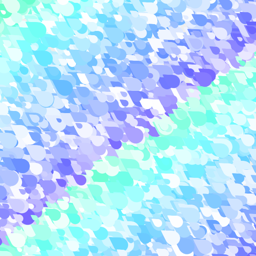
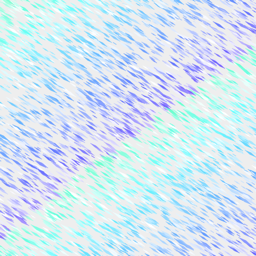
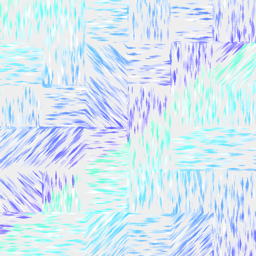
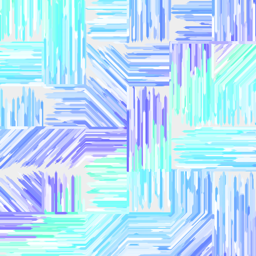
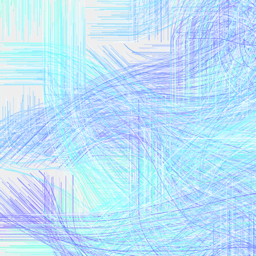
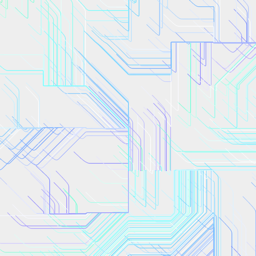
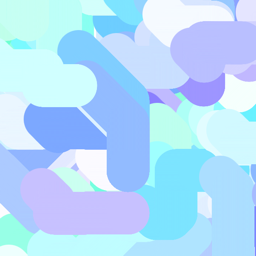
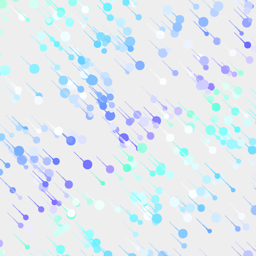
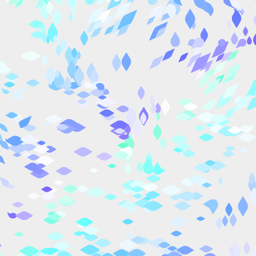
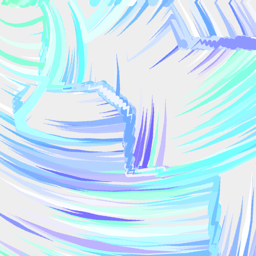
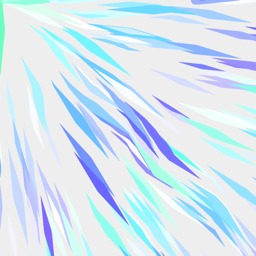
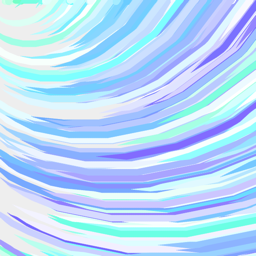
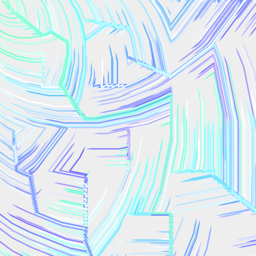
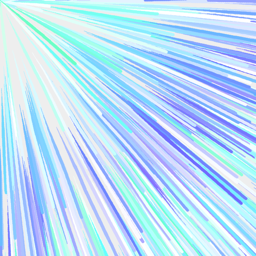
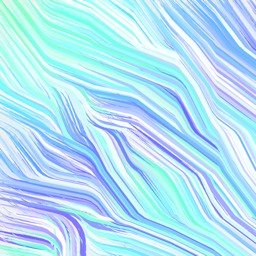
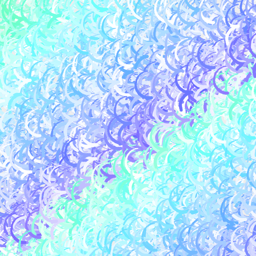
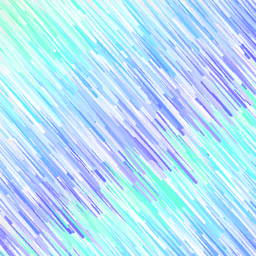
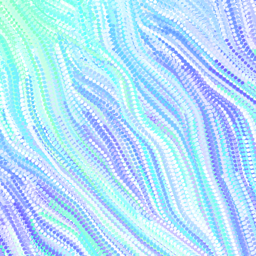
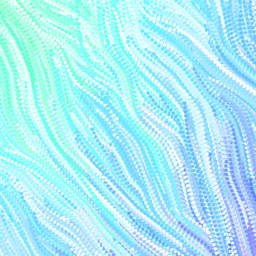
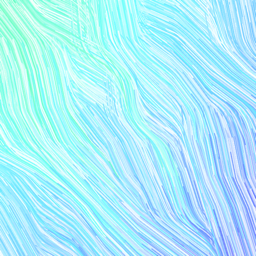
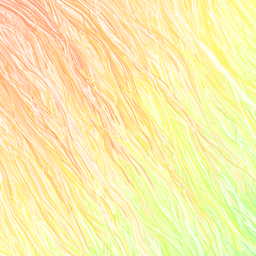
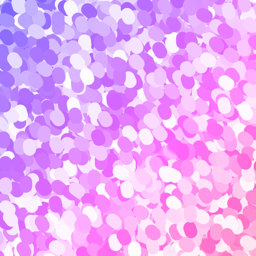
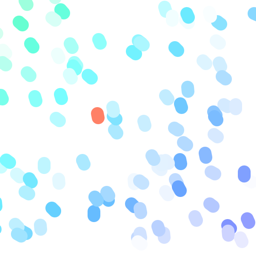
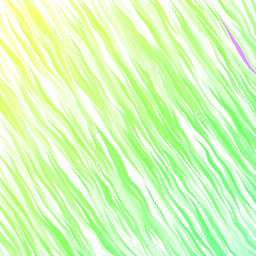
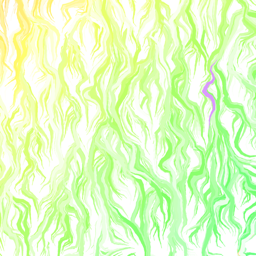
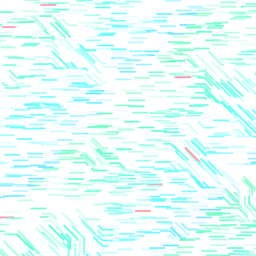
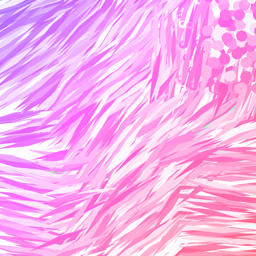
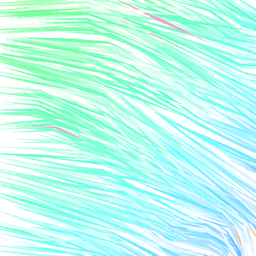
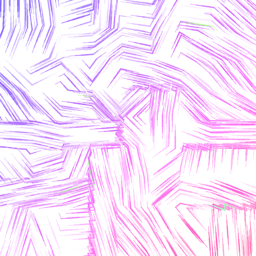